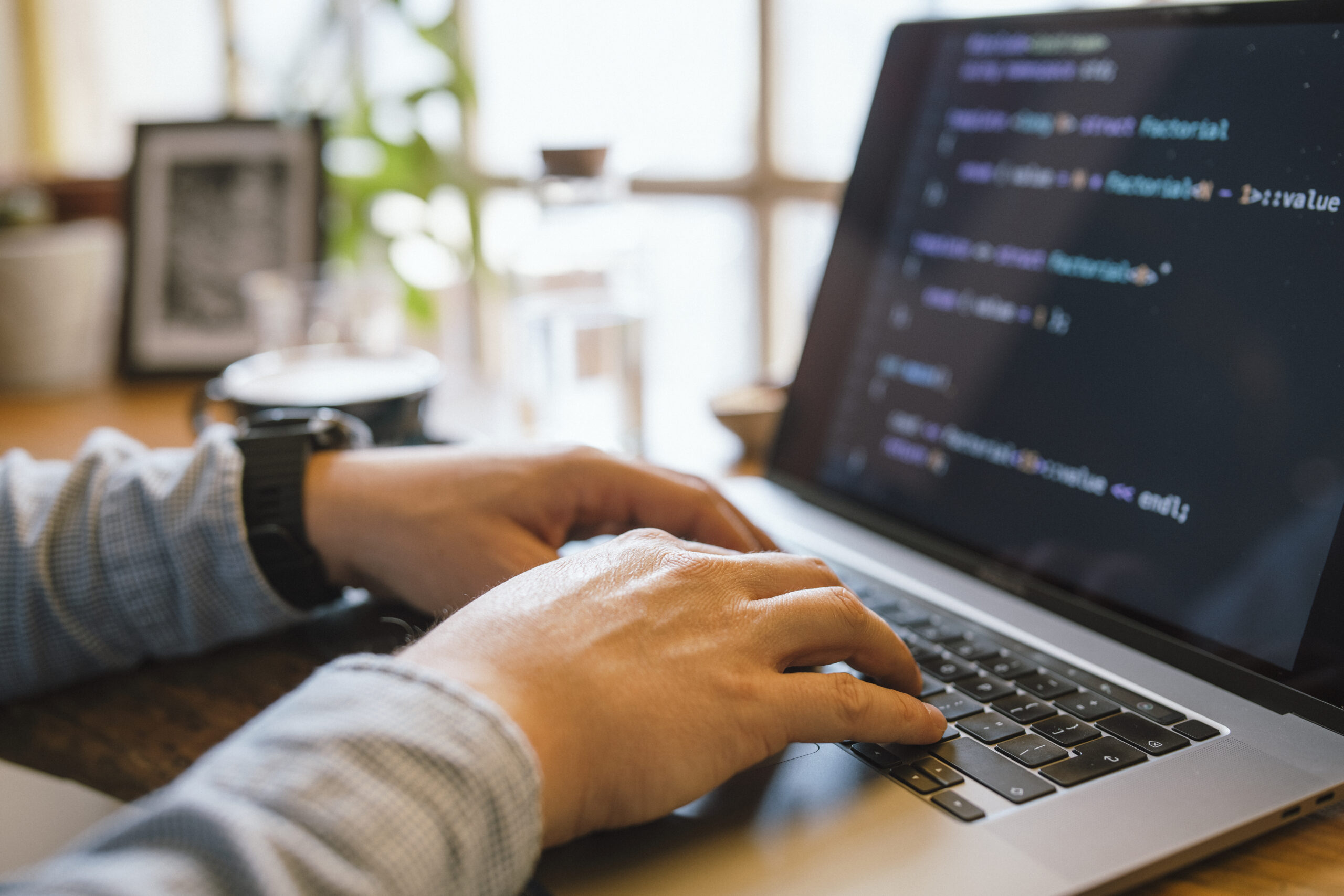
Debugging is The most vital — yet usually overlooked — expertise in a developer’s toolkit. It is not just about repairing broken code; it’s about comprehending how and why matters go Completely wrong, and Studying to Imagine methodically to resolve challenges proficiently. Regardless of whether you are a newbie or simply a seasoned developer, sharpening your debugging capabilities can help save hrs of disappointment and dramatically boost your efficiency. Listed here are many tactics to help you builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Equipment
One of several quickest approaches builders can elevate their debugging competencies is by mastering the resources they use everyday. Even though crafting code is 1 Section of growth, figuring out tips on how to connect with it proficiently through execution is equally crucial. Modern day advancement environments arrive equipped with strong debugging capabilities — but many builders only scratch the area of what these equipment can do.
Take, such as, an Built-in Growth Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools allow you to set breakpoints, inspect the value of variables at runtime, action by code line by line, and in some cases modify code within the fly. When utilized the right way, they Enable you to observe particularly how your code behaves for the duration of execution, which can be a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-end builders. They let you inspect the DOM, observe network requests, watch actual-time performance metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can change disheartening UI troubles into workable duties.
For backend or procedure-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control in excess of operating procedures and memory management. Mastering these tools could possibly have a steeper learning curve but pays off when debugging general performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, turn out to be snug with Model Handle systems like Git to be familiar with code heritage, obtain the exact instant bugs were launched, and isolate problematic variations.
In the end, mastering your instruments means heading beyond default options and shortcuts — it’s about building an intimate familiarity with your improvement surroundings to ensure when difficulties arise, you’re not dropped in the dead of night. The greater you are aware of your applications, the greater time you are able to commit fixing the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the challenge
Among the most essential — and infrequently forgotten — methods in productive debugging is reproducing the situation. Ahead of jumping in to the code or making guesses, builders will need to make a steady atmosphere or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a sport of opportunity, frequently bringing about squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting just as much context as is possible. Inquire questions like: What steps resulted in The difficulty? Which natural environment was it in — advancement, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater detail you've, the a lot easier it gets to isolate the exact conditions underneath which the bug occurs.
When you finally’ve collected enough facts, make an effort to recreate the problem in your neighborhood atmosphere. This may imply inputting the same knowledge, simulating equivalent person interactions, or mimicking method states. If The difficulty appears intermittently, look at creating automatic checks that replicate the edge scenarios or state transitions concerned. These checks not just support expose the problem but in addition prevent regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would materialize only on specific functioning methods, browsers, or beneath unique configurations. Using resources like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It necessitates tolerance, observation, along with a methodical strategy. But as soon as you can continuously recreate the bug, you're currently halfway to repairing it. That has a reproducible state of affairs, you can use your debugging tools more efficiently, examination likely fixes safely and securely, and converse far more clearly along with your crew or consumers. It turns an abstract grievance into a concrete challenge — Which’s where by builders prosper.
Read through and Recognize the Error Messages
Error messages tend to be the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, builders should really master to deal with error messages as direct communications in the procedure. They normally inform you just what happened, where it took place, and often even why it occurred — if you know the way to interpret them.
Start out by looking at the concept carefully As well as in entire. Numerous builders, particularly when below time tension, glance at the very first line and straight away start off creating assumptions. But further inside the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like yahoo — read and fully grasp them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line variety? What module or function activated it? These questions can information your investigation and point you towards the responsible code.
It’s also valuable to understand the terminology with the programming language or framework you’re using. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Finding out to acknowledge these can substantially speed up your debugging method.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context in which the error transpired. Test related log entries, input values, and up to date variations in the codebase.
Don’t forget about compiler or linter warnings both. These normally precede larger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, lessen debugging time, and turn into a additional efficient and confident developer.
Use Logging Properly
Logging is One of the more powerful resources within a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood without having to pause execution or step in the code line by line.
A very good logging system starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts all through enhancement, Details for standard activities (like productive commence-ups), WARN for likely difficulties that don’t split the application, Mistake for genuine troubles, and FATAL when the procedure can’t go on.
Prevent flooding your logs with abnormal or irrelevant facts. An excessive amount logging can obscure crucial messages and slow down your procedure. Center on critical situations, condition modifications, enter/output values, and significant selection details with your code.
Format your log messages Plainly and constantly. Include context, which include timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in output environments in which stepping as a result of code isn’t achievable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, sensible logging is about harmony and clarity. Which has a effectively-assumed-out logging method, you may lessen the time it will take to spot challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not simply a technological activity—it is a sort of investigation. To effectively recognize and take care of bugs, developers should technique the method similar to a detective resolving a secret. This state of mind will help stop working elaborate issues into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency concerns. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate facts as you are able to without having jumping to conclusions. Use logs, check circumstances, and user studies to piece collectively a clear picture of what’s happening.
Next, variety hypotheses. Talk to you: What can be resulting in this habits? Have any alterations not too long ago been created towards the codebase? Has this issue occurred right before underneath related conditions? The objective is to slender down opportunities and recognize possible culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled natural environment. In case you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Pay shut focus to little aspects. Bugs typically hide from the least predicted locations—similar to a missing semicolon, an off-by-just one error, or simply a race issue. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and aid Many others comprehend your reasoning.
By imagining similar to a detective, developers can sharpen their analytical techniques, tactic problems methodically, and grow to be simpler at uncovering concealed issues in sophisticated devices.
Create Exams
Producing checks is one of the most effective strategies to help your debugging skills and All round development efficiency. Tests not just support capture bugs early but will also function a security Web that offers you self-confidence when producing alterations to the codebase. A very well-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Get started with device checks, which deal with individual capabilities or modules. These small, isolated tests can quickly expose whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know the place to search, substantially lowering time invested debugging. Device assessments are In particular valuable for catching regression bugs—concerns that reappear right after previously remaining fastened.
Up coming, integrate integration checks and conclusion-to-conclude exams into your workflow. These help make sure a variety of elements of your software get the job done collectively easily. They’re particularly handy for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which Portion of the pipeline unsuccessful and beneath what circumstances.
Producing exams also forces you to definitely Feel critically regarding your code. To test a aspect appropriately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and observe your take a look at pass when the issue is solved. This approach ensures that precisely the same bug doesn’t return Down the road.
In brief, producing checks turns debugging from a irritating guessing video game right into a structured and predictable procedure—supporting you capture far more bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult difficulty, it’s simple to become immersed in the situation—gazing your monitor for hours, attempting Remedy soon after Alternative. But one of the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for much too long, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you wrote just several hours before. With this condition, your brain turns into significantly less effective at issue-solving. A brief wander, a coffee break, or even switching check here to another endeavor for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious operate inside the track record.
Breaks also help reduce burnout, In particular for the duration of lengthier debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping absent enables you to return with renewed energy and also a clearer frame of mind. You may instantly recognize a missing semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a good general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath tight deadlines, nonetheless it really brings about quicker and simpler debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a sensible strategy. It offers your Mind space to breathe, enhances your standpoint, and assists you stay away from the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Just about every Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a little something beneficial should you make the effort to replicate and review what went Mistaken.
Start out by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit testing, code reviews, or logging? The answers often reveal blind places in the workflow or understanding and assist you to Develop stronger coding routines moving forward.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll start to see styles—recurring difficulties or widespread problems—you can proactively prevent.
In crew environments, sharing Whatever you've discovered from the bug with all your friends could be Particularly powerful. Irrespective of whether it’s via a Slack concept, a brief produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts group performance and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In the end, a lot of the ideal builders will not be those who publish perfect code, but individuals who continuously understand from their errors.
In the long run, each bug you correct provides a fresh layer towards your skill established. So future time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Bettering your debugging techniques requires time, follow, and tolerance — however the payoff is big. It would make you a more effective, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.